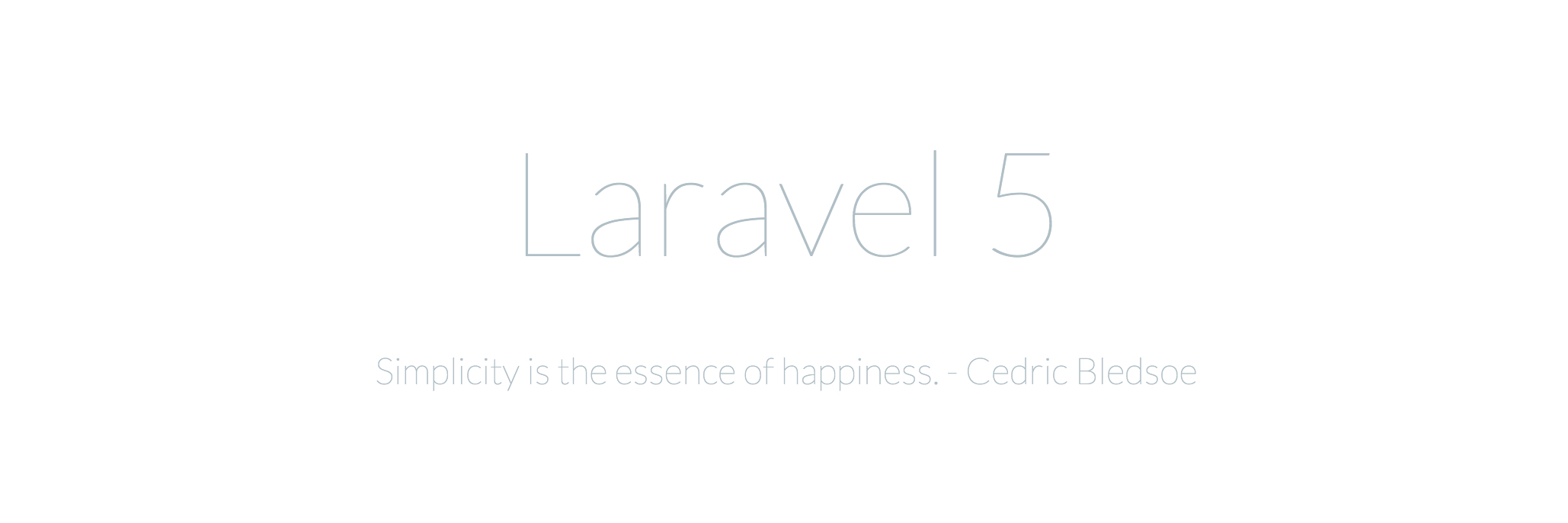
First-time Laravel 5.0 Setup
If you want a video to get you through most of these steps, watch the Laracast.
1. Setup Composer
I already had composer installed, but I need to update it. To update composer, run this command:
1
$ composer self-update
Also, make sure composer is in your
by adding this line to your 1
PATH
1
~/.bash_profile
1
export PATH=~/.composer/vendor/bin:$PATH
2. Install Vagrant
Download vagrant. After installing, you should be able to run
in Terminal and see the command help.1
vagrant
3. Install Virtualbox
Download VirtualBox.
4. Install Homestead
This command takes maybe 5-10 minutes.
1
2
3
$ vagrant box add laravel/homestead
$ composer global require "laravel/homestead=~2.0"
$ homestead init
5. Setup up SSH keys
If you haven’t already setup your SSH keys, run:
1
$ ssh-keygen -t rsa -C "your_email@example.com"
6. Configure Homestead
1
$ homestead edit
- Set
to the directory where all your projects are1
folders: map:
- Set
to1
folders: to:
+ the name of the last directory in1
/home/vagrant/
above1
map:
NOTE: these two folders will automatically always be in sync.
Setup A New Laravel 5.0 Project
Server Setup
1. Configure Project in Homestead
1
$ homestead edit
- Set
to1
sites: map:
1
myproject.app
- Set
to1
sites: to:
1
/home/vagrant/path/to/myproject/public
- Copy the
up top on this file for the next step1
ip:
2. Add Project Url To Hosts File
Add this line to your
file, replacing 1
/etc/hosts
with the IP you copied in the previous step.1
YOUR_IP
1
YOUR_IP myproject.app
3. Run Site Locally
After this command and you should be able to access
in your browser.1
http://myproject.app
1
$ homestead up
Database Access
1. Add Project DB Credentials to Homestead
1
$ homestead edit
- Add to
your project’s database:1
databases:
1
- myproject
- Copy the
up top on this file for the Sequel Pro step1
ip:
2. Configure Database Credentials
Open
file and set the following:1
myproject/.env
1
2
3
4
DB_HOST=127.0.0.1
DB_DATABASE=myproject
DB_USERNAME=homestead
DB_PASSWORD=secret
3. Access Database Via Sequel Pro
Open Sequel Pro and setup a new connection, replacing
with the IP you copied from step 1:1
YOUR_IP
1
2
3
4
5
Name: My Project
Host: YOUR_IP
Username: homestead
Password: secret
Database: myproject
4. Access Database through Terminal
1
2
3
4
$ homestead ssh
$ cd path/to/myproject
$ mysql -uhomestead -p
mysql> show databases;
Laravel 5.0 Project Migration and Seeding
1. Add UserTableSeeder
Add
to 1
UserTableSeeder.php
.1
myproject/databases/seeds/
NOTE: In order to use the
model, I had to add 1
User
to the top of the file.1
use App\User;
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
<?php
use Illuminate\Database\Seeder;
use Illuminate\Database\Eloquent\Model;
use App\User;
class UserTableSeeder extends Seeder {
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
Model::unguard();
DB::table('users')->delete();
User::create([
'name' => 'Your Name',
'email' => 'your@email.com',
'password' => 'your_password'
]);
}
}
2. Edit DatabaseSeeder
Uncomment the following in in
.1
myproject/databases/seeds/DatabaseSeeder.php
1
$this->call('UserTableSeeder');
3. Run Migration and Seeder
1
2
3
$ homestead ssh
$ cd path/to/myproject
$ php artisan migrate:refresh --seed
If that doesn’t work, run
and try again.1
composer dump-autoload
4. Check in DB for tables and values
Yeah… do that ^
Have Questions or Feedback?
Feel free to leave a comment below or tweet me @kevinkirchner.
Go Read Some Documentation
This should get you up and running. If there’s more you need to figure out, read through the laravel docs (http://laravel.com/) and check out laracasts at (https://laracasts.com)